Webhooks
Introduction
Astrada uses webhooks to automatically notify your application when relevant events occur in your account. Webhooks can be utilized as an alternative or complement to direct API requests, in situations where you would prefer to programmatically receive information pushed to you in real-time
In order to receive webhooks, you must select the webhook event types (Event Types) for which you want to receive webhook events, create the webhook and register webhook endpoints where you desire webhooks to be sent.
Creating webhooks
Once you have decided on the appropriate webhook event types for your use case, you can create webhooks using our Create Webhook endpoint. Each webhook requires an HTTPS URL to be registered.
Management and behavior
Astrada will notify your registered webhook URLs as the event happens via a HTTP POST request. The HTTP request payload contains the event object in JSON format. At present, we only support API-based webhook management.
To confirm receipt of a webhook event, your server endpoint should return a 200 OK HTTP status code. If our system detects a failure, it will emit retries in accordance with the table below:
Attempt | Delay | Cumulative Time |
---|---|---|
1 | NA (initial attempt) | -- |
2 | 5s | 5s |
3 | 5m | 5m 5s |
4 | 30m | 35m 5s |
5 | 2h | 2h 35m 5s |
6 | 5h | 7h 35m 5s |
7 | 10h | 17h 35m 5s |
8 | 10h | 27h 35m 5s |
Security and Verification
Each webhook call includes three headers with additional information that are used for verification:
webhook-id
: the unique message identifier for the webhook message. This identifier is unique across all messages but will be the same when the same webhook is being resent.webhook-timestamp
: timestamp in seconds since epoch.webhook-signature
: the Base64 encoded signature composed by concatenating the webhook-id, webhook-timestamp, and body, separated by the full-stop character (.).
The body
is the raw content of the request. The signature is sensitive to any changes, so do not change the body in any way before verifying, as it will cause the signature to be completely different.
Signature Verification
Astrada uses an HMAC with SHA-256 to sign its webhooks.
The signing secret
is only obtained at the creation of the webhook and must be secured as it won’t be visible afterward. For any reason, if you lose access to the secret, we recommend creating a new webhook and deleting the previous one.
To verify the authenticity of the request you should calculate the expected signature and compare it to the one received on the webhook-signature
header. For this, you should HMAC the ${webhook-id}.${webhook-timestamp}.${body}
using your base64 decoded signing secret.
For example, this is how you can calculate the signature in Node.js
const crypto = require('crypto');
const body = ‘{“id”:”random-id”,”other”:”test”}’;
const headers = {
'webhook-id': 'msg_2edtk77s2IbiV6pH2K8KeV2BBza',
'webhook-timestamp': '1712246422',
'webhook-signature': 'v1,qDejq/phQBZBCaw+5Oy/THT0/Xaj8l88JEqPnIqM/aE=',
};
const secret = 'N2ViZDU2ZWMtMGMxYi00NDc5LTgyMTAtZTdjZWUzNmRlZTNh';
const decodedSecret = atob(secret);
const signature = crypto
.createHmac('sha256', decodedSecret)
.update(`${headers['webhook-id']}.${headers['webhook-timestamp']}.${body}`)
.digest('base64');
console.log(signature)
// qDejq/phQBZBCaw+5Oy/THT0/Xaj8l88JEqPnIqM/aE=
This generated signature should match the one sent in the webhook-signature
header.
Make sure to remove the prefix and delimiter (e.g. v1,
) before verifying the signature.
Signature Verification Flow
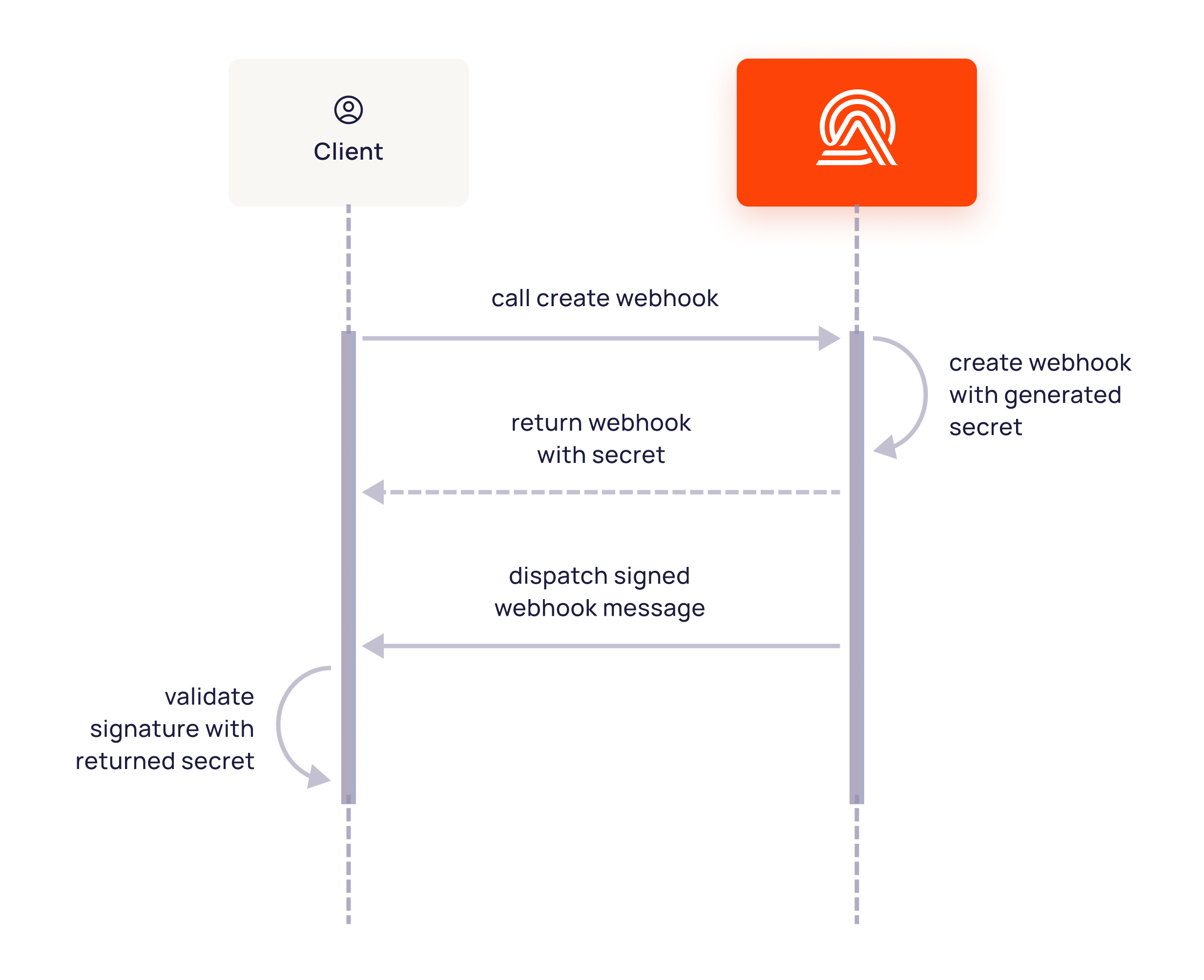
Updated 7 days ago